Note
Go to the end to download the full example code
EFA (Keller and Massart original example)¶
In this example, we perform the Evolving Factor Analysis of a TEST dataset (ref. Keller and Massart, Chemometrics and Intelligent Laboratory Systems, 12 (1992) 209-224 )
import numpy as np
import spectrochempy as scp
Generate a test dataset¶
1) simulated chromatogram¶
t = scp.Coord(np.arange(15), units="minutes", title="time") # time coordinates
c = scp.Coord(range(2), title="components") # component coordinates
data = np.zeros((2, 15), dtype=np.float64)
data[0, 3:8] = [1, 3, 6, 3, 1] # compound 1
data[1, 5:11] = [1, 3, 5, 3, 1, 0.5] # compound 2
dsc = scp.NDDataset(data=data, coords=[c, t])
dsc.plot(title="concentration")
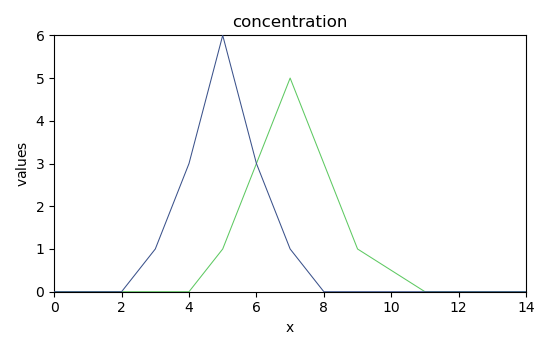
<_Axes: title={'center': 'concentration'}, xlabel='x', ylabel='values $\\mathrm{}$'>
2) absorption spectra¶
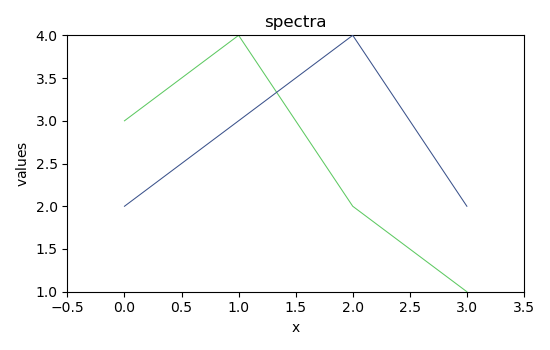
<_Axes: title={'center': 'spectra'}, xlabel='x', ylabel='values $\\mathrm{}$'>
3) simulated data matrix¶
dataset = scp.dot(dsc.T, dss)
dataset.data = np.random.normal(dataset.data, 0.1)
dataset.title = "intensity"
dataset.plot(title="calculated dataset")
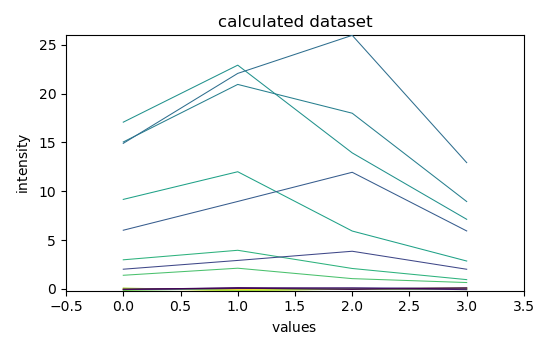
<_Axes: title={'center': 'calculated dataset'}, xlabel='values $\\mathrm{}$', ylabel='intensity $\\mathrm{}$'>
4) evolving factor analysis (EFA)¶
<spectrochempy.analysis.decomposition.efa.EFA object at 0x7f5231755240>
Plots of the log(EV) for the forward and backward analysis
efa.f_ev.T.plot(yscale="log", legend=efa.f_ev.k.labels)
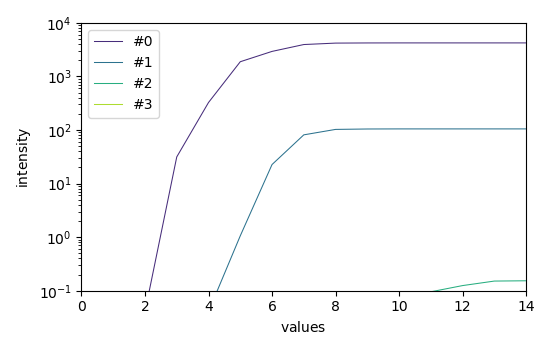
<_Axes: xlabel='values $\\mathrm{}$', ylabel='intensity $\\mathrm{}$'>
efa.b_ev.T.plot(yscale="log", legend=efa.b_ev.k.labels)
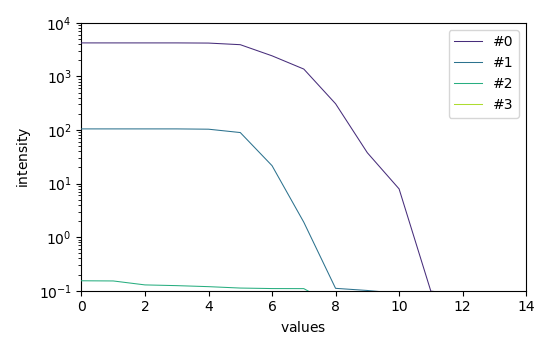
<_Axes: xlabel='values $\\mathrm{}$', ylabel='intensity $\\mathrm{}$'>
Looking at these EFA curves, it is quite obvious that only two components are really significant, and this corresponds to the data that we have in input. We can consider that the third EFA components is mainly due to the noise, and so we can use it to set a cut of values
we concatenate the datasets to plot them in a single figure
both = scp.concatenate(f2, b2)
both.T.plot(yscale="log")
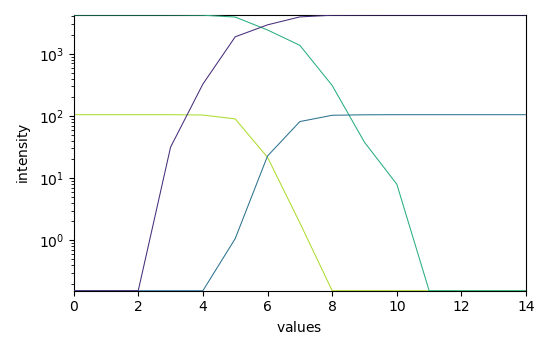
/home/runner/micromamba/envs/scpy_docs/lib/python3.10/site-packages/spectrochempy/processing/transformation/concatenate.py:196: UserWarning: Some dataset(s) coordinates in the k dimension are None.
warn(f"Some dataset(s) coordinates in the {dim} dimension are None.")
<_Axes: xlabel='values $\\mathrm{}$', ylabel='intensity $\\mathrm{}$'>
Get the abstract concentration profile based on the FIFO EFA analysis
C = efa.transform()
C.T.plot(title="EFA concentration")
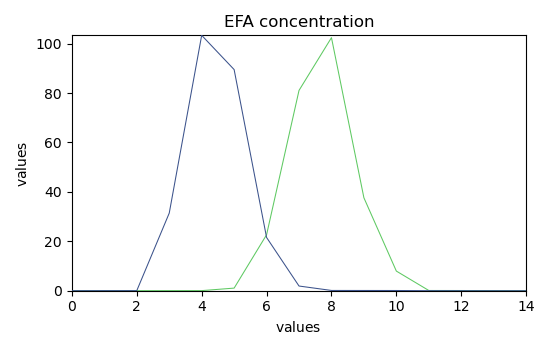
<_Axes: title={'center': 'EFA concentration'}, xlabel='values $\\mathrm{}$', ylabel='values $\\mathrm{}$'>
This ends the example ! The following line can be uncommented if no plot shows when running the .py script with python
# scp.show()
Total running time of the script: ( 0 minutes 1.152 seconds)