spectrochempy.read¶
- read(*paths, **kwargs)[source]¶
Generic read method.
This method is generally able to load experimental files based on extensions.
- Parameters
*paths (
str
,Path
object objects or valid urls, optional) – The data source(s) can be specified by the name or a list of name for the file(s) to be loaded:e.g., ( filename1, filename2, …, **kwargs )*
If the list of filenames are enclosed into brackets:
e.g., ( [ filename1, filename2, … ], **kwargs )
The returned datasets are merged to form a single dataset, except if
merge
is set toFalse
.If a source is not provided (i.e., no
paths
, norcontent
), a dialog box will be opened to select files.**kwargs (keyword parameters, optional) – See Other Parameters.
- Returns
object (
NDDataset
or list ofNDDataset
) – The returned dataset(s).- Other Parameters
protocol (
str
, optional) –Protocol
used for reading. It can be one of {'scp'
,'omnic'
,'opus'
,'topspin'
,'matlab'
,'jcamp'
,'csv'
,'excel'
}. If not provided, the correct protocol is inferred (whenever it is possible) from the filename extension.directory (
Path
object objects or valid urls, optional) – From where to read the files.merge (
bool
, optional, default:False
) – IfTrue
and several filenames or adirectory
have been provided as arguments, then a singleNDDataset
with merged (stacked along the first dimension) is returned.sortbydate (
bool
, optional, default:True
) – Sort multiple filename by acquisition date.description (
str
, optional) – A Custom description.origin (one of {
'omnic'
,'tga'
}, optional) – Used when reading with the CSV protocol. In order to properly interpret CSV file it can be necessary to set the origin of the spectra. Up to now only'omnic'
and'tga'
have been implemented.csv_delimiter (
str
, optional, default:csv_delimiter
) – Set the column delimiter in CSV file.content (
bytes
object, optional) – Instead of passing a filename for further reading, a bytes content can be directly provided as bytes objects. The most convenient way is to use a dictionary. This feature is particularly useful for a GUI Dash application to handle drag and drop of files into a Browser.iterdir (
bool
, optional, default:True
) – IfTrue
and no filename was provided, all files present in the provideddirectory
are returned (and merged ifmerge
isTrue
. It is assumed that all the files correspond to current reading protocol.Changed in version 0.6.2:
iterdir
replace the deprecatedlistdir
argument.recursive (
bool
, optional, default:False
) – Read also in subfolders.replace_existing (
bool
, optional, default:False
) – Used only when url are specified. By default, existing files are not replaced so not downloaded.download_only (
bool
, optional, default:False
) – Used only when url are specified. If True, only downloading and saving of the files is performed, with no attempt to read their content.read_only (
bool
, optional, default:True
) – Used only when url are specified. If True, saving of the files is performed in the current directory, or in the directory specified by the directory parameter.
See also
read_zip
Read Zip archives (containing spectrochempy readable files)
read_dir
Read an entire directory.
read_opus
Read OPUS spectra.
read_labspec
Read Raman LABSPEC spectra (
.txt
).read_omnic
Read Omnic spectra (
.spa
,.spg
,.srs
).read_soc
Read Surface Optics Corps. files (
.ddr
,.hdr
or.sdr
).read_galactic
Read Galactic files (
.spc
).read_quadera
Read a Pfeiffer Vacuum’s QUADERA mass spectrometer software file.
read_topspin
Read TopSpin Bruker NMR spectra.
read_csv
Read CSV files (
.csv
).read_jcamp
Read Infrared JCAMP-DX files (
.jdx
,.dx
).read_matlab
Read Matlab files (
.mat
,.dso
).read_carroucell
Read files in a directory after a carroucell experiment.
read_wire
Read REnishaw Wire files (
.wdf
).
Examples
Reading a single OPUS file (providing a windows type filename relative to the default
datadir
)>>> scp.read('irdata\\OPUS\\test.0000') NDDataset: [float64] a.u. (shape: (y:1, x:2567))
Reading a single OPUS file (providing a unix/python type filename relative to the default
datadir
) Note that here read_opus is called as a classmethod of the NDDataset class>>> scp.read('irdata/OPUS/test.0000') NDDataset: [float64] a.u. (shape: (y:1, x:2567))
Single file specified with pathlib.Path object
>>> from pathlib import Path >>> folder = Path('irdata/OPUS') >>> p = folder / 'test.0000' >>> scp.read(p) NDDataset: [float64] a.u. (shape: (y:1, x:2567))
Multiple files not merged (return a list of datasets). Note that a directory is specified
>>> le = scp.read('test.0000', 'test.0001', 'test.0002', directory='irdata/OPUS') >>> len(le) 3 >>> le[0] NDDataset: [float64] a.u. (shape: (y:1, x:2567))
Multiple files merged as the
merge
keyword is set to true>>> scp.read('test.0000', 'test.0001', 'test.0002', directory='irdata/OPUS', merge=True) NDDataset: [float64] a.u. (shape: (y:3, x:2567))
Multiple files to merge : they are passed as a list instead of using the keyword
merge
>>> scp.read(['test.0000', 'test.0001', 'test.0002'], directory='irdata/OPUS') NDDataset: [float64] a.u. (shape: (y:3, x:2567))
Multiple files not merged : they are passed as a list but
merge
is set to false>>> le = scp.read(['test.0000', 'test.0001', 'test.0002'], directory='irdata/OPUS', merge=False) >>> len(le) 3
Read without a filename. This has the effect of opening a dialog for file(s) selection
>>> nd = scp.read()
Read in a directory (assume that only OPUS files are present in the directory (else we must use the generic
read
function instead)>>> le = scp.read(directory='irdata/OPUS') >>> len(le) 2
Again we can use merge to stack all 4 spectra if thet have compatible dimensions.
>>> scp.read(directory='irdata/OPUS', merge=True) [NDDataset: [float64] a.u. (shape: (y:1, x:5549)), NDDataset: [float64] a.u. (shape: (y:4, x:2567))]
Examples using spectrochempy.read
¶

Using plot_multiple to plot several datasets on the same figure
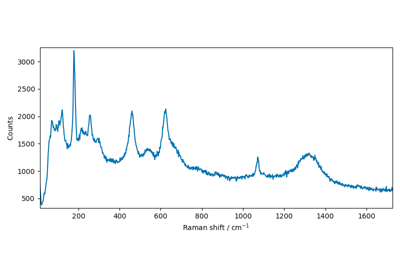
Savitky-Golay and Whittaker-Eilers smoothing of a Raman spectrum