spectrochempy.Filter¶
- class Filter(log_level='WARNING', *, cval=0.0, delta=1.0, deriv=0, lamb=1.0, method='savgol', mode='interp', order=2, size=5)[source]¶
Filters/smoothers processor.
The filters can be applied to 1D datasets consisting in a single row with n_features or to a 2D dataset with shape (n_observations, n_features).
Various filters/smoothers can be applied to the data. The currently available filters are:
- Parameters
log_level (any of [
"INFO"
,"DEBUG"
,"WARNING"
,"ERROR"
], optional, default:"WARNING"
) – The log level at startup. It can be changed later on using theset_log_level
method or by changing thelog_level
attribute.cval (
float
, optional, default: 0.0) – Value to fill past the edges of the input ifmode
is ‘constant’.delta (
float
, optional, default: 1.0) – The spacing of the samples to which the filter will be applied. This is only used if deriv > 0.deriv (
int
, optional, default: 0) – The order of the derivative to compute in the case of the Savitzky-Golay (savgol) filter. This must be a non-negative integer. The default is 0, which means to filter the data without differentiating.lamb (
float
, optional, default: 1.0) – Smoothing/Regularization parameter. The largerlamb
, the smoother the data.method (any value of [
'avg'
,'han'
,'hamming'
,'bartlett'
,'blackman'
,'median'
,'savgol'
,'whittaker'
], optional, default:'savgol'
) – The filter method to be applied. By default, the Savitzky-Golay (savgol) filter is applied.mode (any value of [
'mirror'
,'constant'
,'nearest'
,'wrap'
,'interp'
], optional, default:'interp'
) – The type of extension to use for the padded signal to which the filter is applied.When mode is ‘constant’, the padding value is given by
cval
.When the ‘interp’ mode is selected (the default), no extension is used. Instead, a polynomial of degree
order
is fit to the lastsize
values of the edges, and this polynomial is used to evaluate the last window_length // 2 output values.When mode is ‘nearest’, the last size values are repeated.
When mode is ‘mirror’, the padding is created by reflecting the signal about the end of the signal.
When mode is ‘wrap’, the signal is wrapped around on itself to create the padding.
See
scipy.signal.savgol_filter
for more details on ‘mirror’, ‘constant’, ‘wrap’, and ‘nearest’.order (
int
, optional, default: 2) – The order of the polynomial used to fit the datain the case of the Savitzky-Golay (savgol) filter.order
must be less than size. In the case of the Whittaker-Eilers filter, order is the difference order of the penalized least squares.size (
positiveoddinteger
, optional, default: 5) – The size of the filter window.size must be a positive odd integer.
See also
smooth
Function to smooth data using various window filters.
savgol
Savitzky-Golay filter.
savgol_filter
Alias of
savgol
whittaker
Whittaker-Eilers filter.
Attributes Summary
traitlets.config.Config
object.Value to fill past the edges of the input if
mode
is ‘constant’.The spacing of the samples to which the filter will be applied.
The order of the derivative to compute in the case of the Savitzky-Golay (savgol) filter.
Smoothing/Regularization parameter.
Return
log
output.The filter method to be applied.
The type of extension to use for the padded signal to which the filter is applied.
Object name
The order of the polynomial used to fit the datain the case of the Savitzky-Golay (savgol) filter.
The size of the filter window.size must be a positive odd integer.
Methods Summary
parameters
([replace, removed, default])Alias for
params
method.params
([default])Current or default configuration values.
reset
()Reset configuration parameters to their default values
to_dict
()Return config value in a dict form.
transform
(dataset[, dim])Transform the input dataset X using the current model.
Attributes Documentation
- config¶
traitlets.config.Config
object.
- delta¶
The spacing of the samples to which the filter will be applied. This is only used if deriv > 0.
- deriv¶
The order of the derivative to compute in the case of the Savitzky-Golay (savgol) filter. This must be a non-negative integer. The default is 0, which means to filter the data without differentiating.
- log¶
Return
log
output.
- method¶
The filter method to be applied. By default, the Savitzky-Golay (savgol) filter is applied.
- mode¶
The type of extension to use for the padded signal to which the filter is applied.
When mode is ‘constant’, the padding value is given by
cval
.When the ‘interp’ mode is selected (the default), no extension is used. Instead, a polynomial of degree
order
is fit to the lastsize
values of the edges, and this polynomial is used to evaluate the last window_length // 2 output values.When mode is ‘nearest’, the last size values are repeated.
When mode is ‘mirror’, the padding is created by reflecting the signal about the end of the signal.
When mode is ‘wrap’, the signal is wrapped around on itself to create the padding.
See
scipy.signal.savgol_filter
for more details on ‘mirror’, ‘constant’, ‘wrap’, and ‘nearest’.
- name¶
Object name
- order¶
The order of the polynomial used to fit the datain the case of the Savitzky-Golay (savgol) filter.
order
must be less than size. In the case of the Whittaker-Eilers filter, order is the difference order of the penalized least squares.
- size¶
The size of the filter window.size must be a positive odd integer.
Methods Documentation
- parameters(replace="params", removed="0.7.1") def parameters(self, default=False)[source]¶
Alias for
params
method.
- transform(dataset, dim=-1)¶
Transform the input dataset X using the current model.
- Parameters
dataset (
NDDataset
or array-like of shape (n_observations
,n_features
)) – Input data, where n_observations is the number of observations and n_features is the number of features.dim (
int
orstr
, optional, default: -1,) – Dimension along which the method is applied. By default, the method is applied to the last dimension. Ifdim
is specified as an integer it is equivalent to the usualaxis
numpy parameter.
- Returns
NDDataset
– The transformed dataset.
Examples using spectrochempy.Filter
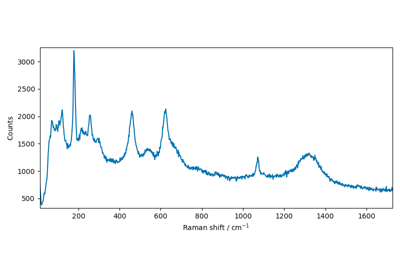
Savitky-Golay and Whittaker-Eilers smoothing of a Raman spectrum